The Ultimate Guide to Outstanding NestJS Core Features
Hurry up and boost your energy with this great guide to understanding and implementing NestJS core features. Get started today and see the results.
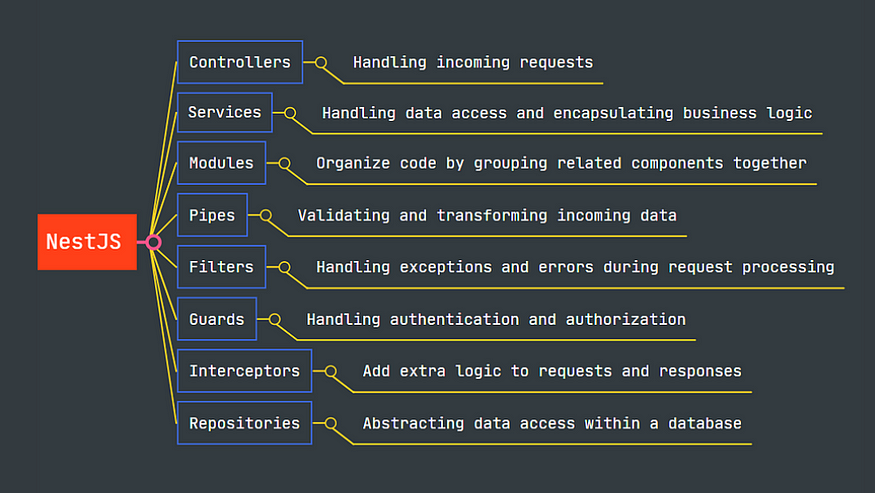
NestJS has emerged as a leading framework for building robust server-side applications, admired by developers for its scalability and efficiency. Understanding the framework’s core components is crucial for mastering NestJS development and creating well-structured applications that stand the test of time.
In this comprehensive guide, we’ll delve into the fundamental components of NestJS: Controllers, Services, Modules, Pipes, Filters, Guards, Interceptors, and Repositories. By the end of this journey, you’ll have a solid understanding of how each piece fits into the NestJS puzzle and how to leverage them effectively in your projects.
Let’s break each one down with an example.
1. Controllers
Controllers serve as the entry points for incoming requests in a NestJS application. They handle request routing and are responsible for invoking the appropriate service methods to process and respond to client requests. By separating concerns and keeping the request handling logic within controllers, NestJS promotes clean and maintainable codebases.
@Controller('cats')
export class CatsController {
constructor(private readonly catsService: CatsService) {}
@Get()
findAll(): Promise<Cat[]> {
return this.catsService.findAll();
}
@Post()
create(@Body() createCatDto: CreateCatDto): Promise<Cat> {
return this.catsService.create(createCatDto);
}
}
In the example provided, we have a CatsController
class decorated with @Controller('cats')
. This decorator specifies the base route for all routes defined within the controller. Within the controller, we define methods like findAll()
and create()
, which handle GET and POST requests, respectively. These methods delegate the actual logic to a CatsService
instance injected into the controller.
2. Services
Services encapsulate business logic and data access operations within a NestJS application. They are responsible for interacting with databases, external APIs, or any other data sources, abstracting away the implementation details from controllers. By promoting code reuse and separation of concerns, services play a crucial role in building maintainable and testable applications.
@Injectable()
export class CatsService {
constructor(@InjectRepository(Cat) private readonly catRepository: Repository<Cat>) {}
findAll(): Promise<Cat[]> {
return this.catRepository.find();
}
create(createCatDto: CreateCatDto): Promise<Cat> {
const cat = this.catRepository.create(createCatDto);
return this.catRepository.save(cat);
}
}
The CatsService
class is responsible for encapsulating business logic related to cats in our application. It is decorated with @Injectable()
to indicate that it can be injected into other components, such as controllers. Within the service, we define methods like findAll()
and create()
that interact with a data source (e.g., a database) to retrieve or manipulate cat data.
3. Modules
Modules are used to organize the application into cohesive units of functionality. They encapsulate related controllers, services, and other providers, promoting modularity and reusability. NestJS employs a modular architecture, allowing developers to structure their applications into feature modules, which can be easily plugged into or removed from the application.
@Module({
imports: [TypeOrmModule.forFeature([Cat])],
controllers: [CatsController],
providers: [CatsService],
})
export class CatsModule {}
The CatsModule
class is a feature module that encapsulates the cats-related components of our application, including the CatsController
and CatsService
. By defining the module, we can import it into the root module or other feature modules, making it easy to organize and maintain our application’s codebase.
4. Pipes
Pipes enable data transformation and validation within NestJS applications. They can be used to sanitize and validate incoming data before it reaches the controller, ensuring data integrity and security. NestJS provides built-in pipes for common use cases, and developers can also create custom pipes tailored to their application’s specific requirements.
@Injectable()
export class ValidationPipe implements PipeTransform {
transform(value: any, metadata: ArgumentMetadata) {
// Validation logic here
return value;
}
}
The ValidationPipe
class demonstrates how pipes can be used for data validation within NestJS applications. In this example, we define a custom pipe that implements the PipeTransform
interface. Within the transform()
method, we perform validation logic on the incoming data and return the transformed value if validation passes.
5. Filters
Filters intercept exceptions and errors that occur during request processing and allow developers to customize error handling behavior. They provide a centralized mechanism for handling errors and responding to clients with appropriate HTTP status codes and error messages. Filters can be applied globally or scoped to specific controllers or routes.
@Catch(HttpException)
export class HttpExceptionFilter implements ExceptionFilter {
catch(exception: HttpException, host: ArgumentsHost) {
const ctx = host.switchToHttp();
const response = ctx.getResponse<Response>();
const status = exception.getStatus();
response.status(status).json({
statusCode: status,
message: 'Internal Server Error',
});
}
}
The HttpExceptionFilter
class illustrates how filters can be used to handle exceptions and errors in NestJS applications. In this example, we define a filter that catches instances of HttpException
and returns an appropriate error response to the client, including the status code and a generic error message.
6. Guards
Guards are used for authentication and authorization purposes in NestJS applications. They intercept incoming requests and determine whether the request should be processed further based on certain conditions, such as the user’s authentication status or role. Guards are essential for securing routes and protecting sensitive resources from unauthorized access.
@Injectable()
export class AuthGuard implements CanActivate {
canActivate(context: ExecutionContext): boolean | Promise<boolean> | Observable<boolean> {
const request = context.switchToHttp().getRequest();
return request.isAuthenticated();
}
}
The AuthGuard
class showcases how guards can be used for authentication purposes in NestJS applications. In this example, we implement the CanActivate
interface to create a guard that checks whether the user is authenticated before allowing access to protected routes.
7. Interceptors
Interceptors provide a way to intercept incoming requests and outgoing responses, allowing developers to inject additional logic before or after request processing. They can be used for tasks such as logging, error handling, data transformation, or adding custom headers to responses. Interceptors offer a flexible and powerful mechanism for extending the functionality of NestJS applications.
@Injectable()
export class LoggingInterceptor implements NestInterceptor {
intercept(context: ExecutionContext, next: CallHandler): Observable<any> {
console.log('Before...');
const now = Date.now();
return next.handle().pipe(tap(() => console.log(`After... ${Date.now() - now}ms`)));
}
}
The LoggingInterceptor
class demonstrates how interceptors can be used to add extra logic to requests and responses in NestJS applications. In this example, we create an interceptor that logs the duration of each request processing, providing insight into the performance of our application.
8. Repositories
Repositories abstract data access operations within a NestJS application and provide a clean interface for interacting with databases. They encapsulate CRUD (Create, Read, Update, Delete) operations and enable developers to work with domain objects without directly dealing with database-specific details. NestJS integrates seamlessly with ORM libraries like TypeORM, making database interactions straightforward and efficient.
@Injectable()
export class CatsRepository {
constructor(private readonly catRepository: Repository<Cat>) {}
findAll(): Promise<Cat[]> {
return this.catRepository.find();
}
create(createCatDto: CreateCatDto): Promise<Cat> {
const cat = this.catRepository.create(createCatDto);
return this.catRepository.save(cat);
}
}
The CatsRepository
class exhibits how repositories can abstract data access operations within NestJS applications. In this example, we define a repository class that encapsulates CRUD operations for cat entities, allowing us to interact with the underlying database without exposing its implementation details throughout the application.
Conclusion:
Mastering the core components of NestJS is essential for building efficient and scalable server-side applications. By understanding how controllers, services, modules, pipes, filters, guards, interceptors, and repositories work together, developers can leverage the full power of NestJS to create robust and maintainable codebases.
As you continue your journey with NestJS, remember to explore the documentation and experiment with different patterns and best practices to elevate your development skills further.